A Comprehensive Guide to MySQL Joins
In the realm of relational databases, joins are an essential feature that allows users to retrieve data from multiple tables in a single query. MySQL, one of the most popular database management systems, provides robust support for various types of joins. Understanding how to use joins effectively can significantly enhance your ability to query and manipulate your data. In this blog post, we’ll explore the different types of MySQL joins, their syntax, practical use cases, and guidance on when to use each type of join.
What is a Join?
A join is a SQL operation performed on two or more tables to retrieve data based on a related column between them. Joins are used to combine rows from two or more tables, creating a set that can be used for further analysis or reporting.
Why Use Joins?
Joins are used to:
- Retrieve related data from multiple tables: Often, data is spread across multiple tables to reduce redundancy and ensure data integrity. Joins help in combining this related data.
- Simplify complex queries: By using joins, you can fetch all necessary data in a single query, reducing the need for multiple queries and data processing in your application.
- Improve performance: Properly written join queries can be more efficient and faster compared to fetching data separately and then combining it.
Types of Joins in MySQL
MySQL supports several types of joins:
- Inner Join
- Left Join (or Left Outer Join)
- Right Join (or Right Outer Join)
- Full Join (or Full Outer Join)
- Cross Join
- Self Join
Inner Join
An Inner Join returns records that have matching values in both tables. It’s the most common type of join used in queries.
SELECT columns
FROM table1
INNER JOIN table2
ON table1.column = table2.column;
Example:
Consider two tables, employees
and departments
. To find employees along with their department names:
SELECT employees.name, departments.name
FROM employees
INNER JOIN departments
ON employees.department_id = departments.id;
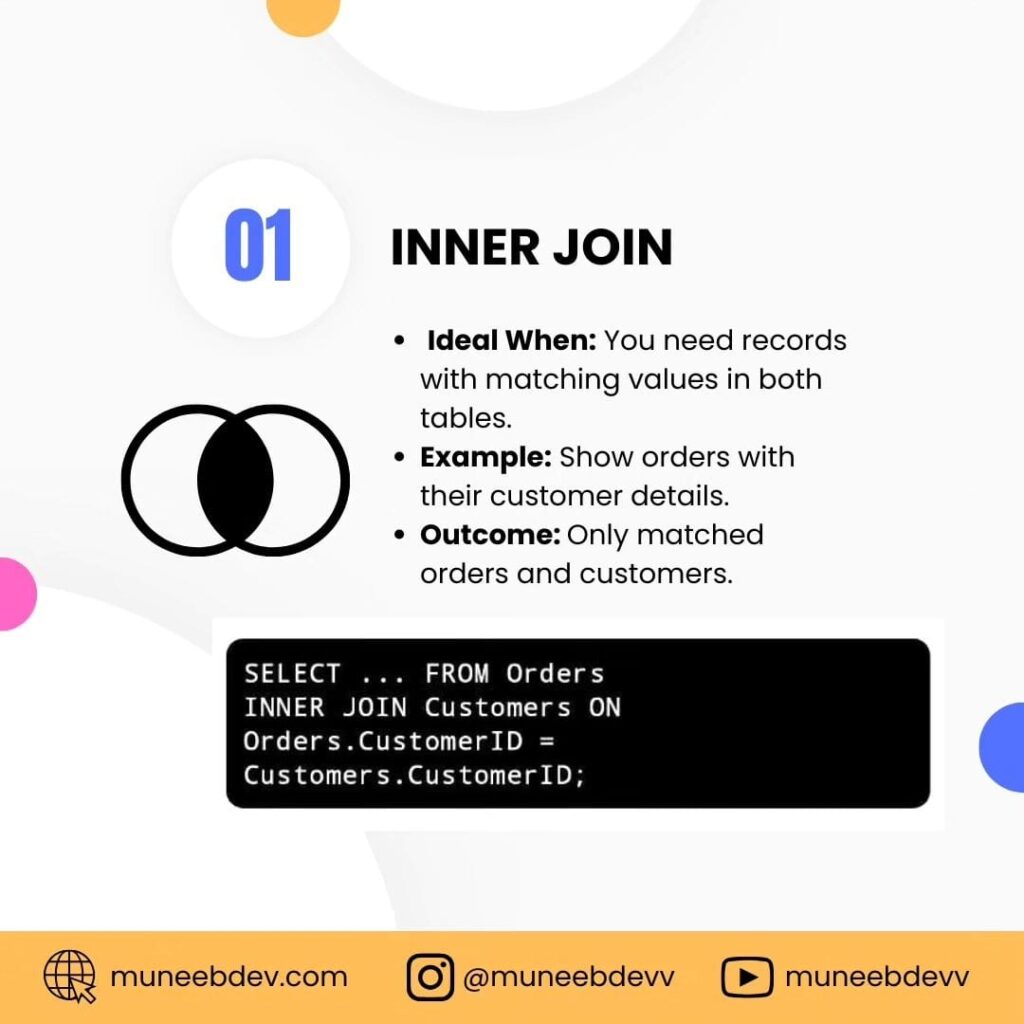
When to Use Inner Join:
- When you need to retrieve records that have matching values in both tables.
- Example: Finding employees who are assigned to a department.
Left Join
A Left Join returns all records from the left table and the matched records from the right table. If no match is found, NULL values are returned for columns from the right table.
Syntax:
SELECT columns
FROM table1
LEFT JOIN table2
ON table1.column = table2.column;
Example:
To find all employees and their department names, even if some employees are not assigned to any department:
SELECT employees.name, departments.name
FROM employees
LEFT JOIN departments
ON employees.department_id = departments.id;
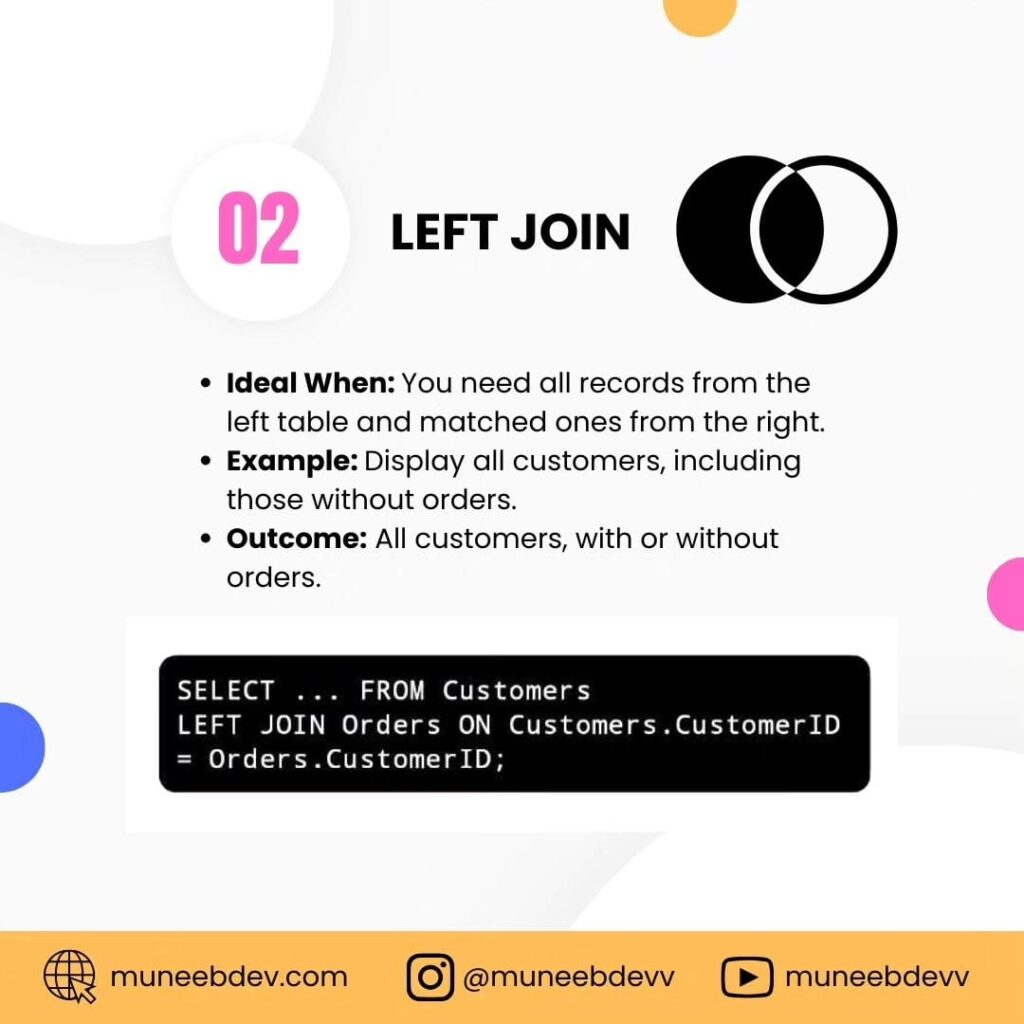
When to Use Left Join:
- When you need all records from the left table, regardless of whether there is a match in the right table.
- Example: Listing all employees and their departments, including those not assigned to any department.
Right Join
A Right Join returns all records from the right table and the matched records from the left table. If no match is found, NULL values are returned for columns from the left table.
Syntax:
SELECT columns
FROM table1
RIGHT JOIN table2
ON table1.column = table2.column;
Example:
To find all departments and their employees, even if some departments have no employees:
SELECT employees.name, departments.name
FROM employees
RIGHT JOIN departments
ON employees.department_id = departments.id;
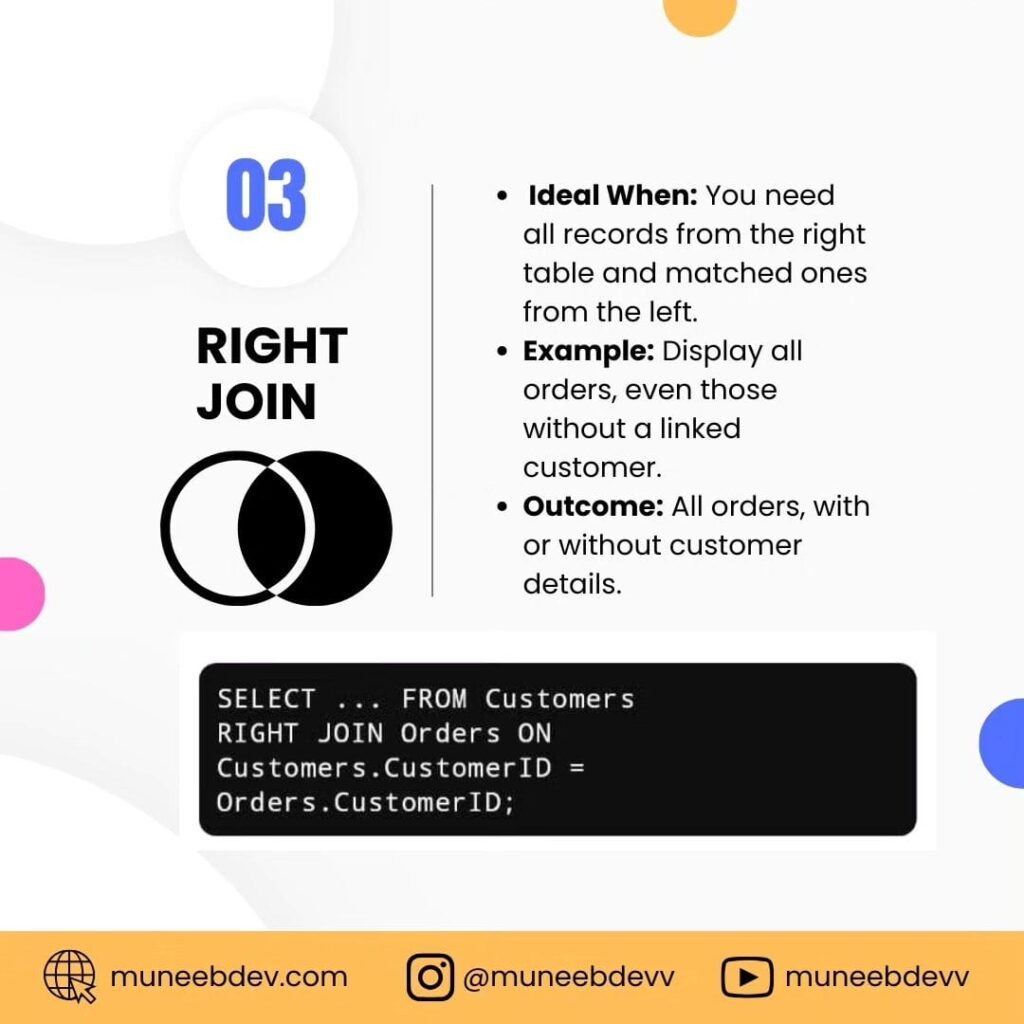
When to Use Right Join:
- When you need all records from the right table, regardless of whether there is a match in the left table.
- Example: Listing all departments and their employees, including departments without employees.
Full Join
A Full Join returns all records when there is a match in either left or right table records. If there is no match, the result is NULL from the side that does not have a match.
Syntax:
MySQL does not directly support FULL JOIN, but you can achieve the same result using a combination of LEFT JOIN and RIGHT JOIN with UNION.
Example:
SELECT employees.name, departments.name
FROM employees
LEFT JOIN departments
ON employees.department_id = departments.id
UNION
SELECT employees.name, departments.name
FROM employees
RIGHT JOIN departments
ON employees.department_id = departments.id;
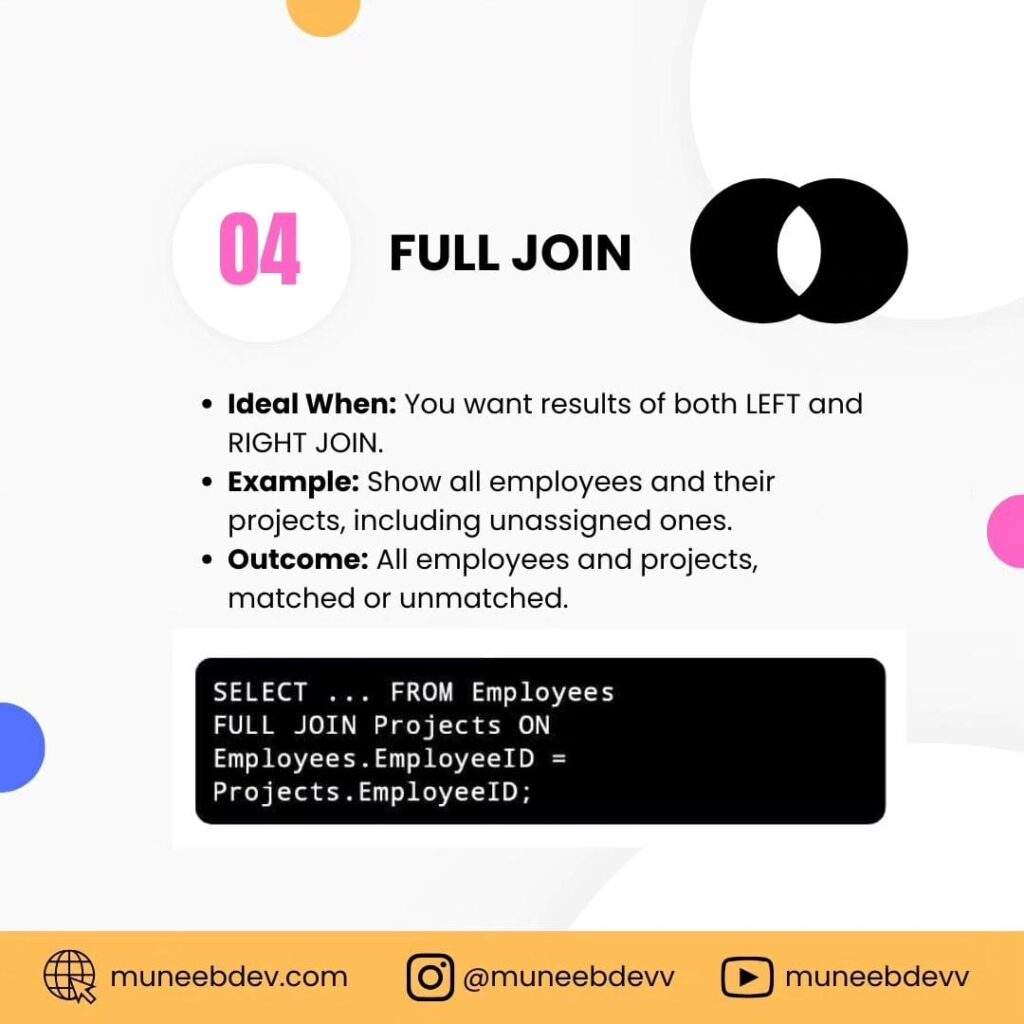
When to Use Full Join:
- When you need all records from both tables, with NULLs in place where there is no match.
- Example: Listing all employees and departments, showing all combinations of employees and departments.
Cross Join
A Cross Join returns the Cartesian product of the two tables, meaning it combines all rows of the first table with all rows of the second table.
Syntax:
SELECT columns
FROM table1
CROSS JOIN table2;
Example:
To combine every employee with every department:
SELECT employees.name, departments.name
FROM employees
CROSS JOIN departments;
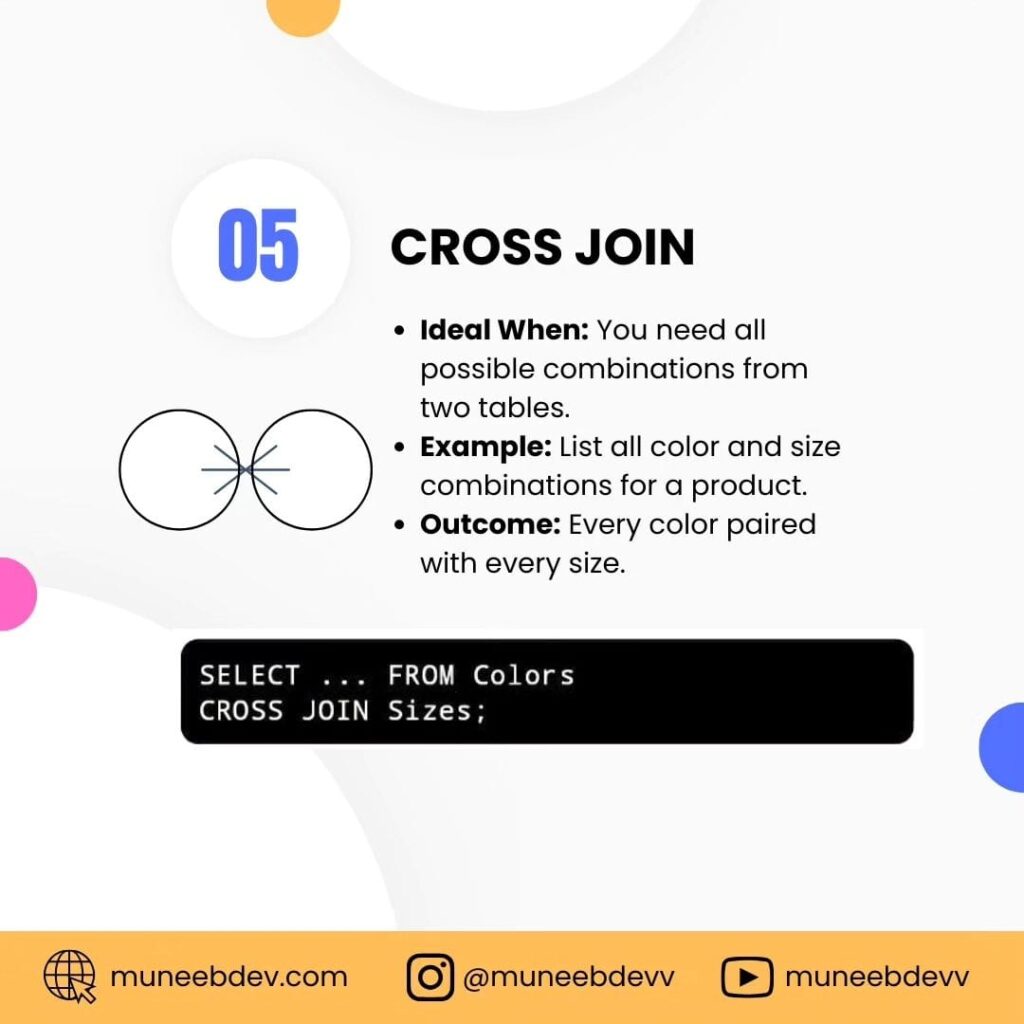
When to Use Cross Join:
- When you need all possible combinations of rows between two tables.
- Example: Generating all possible pairings of employees and departments for analysis.
Self Join
A Self Join is a regular join but the table is joined with itself. It’s useful for comparing rows within the same table.
Syntax:
SELECT a.columns, b.columns
FROM table a, table b
WHERE condition;
Example:
To find pairs of employees who work in the same department:
SELECT a.name AS Employee1, b.name AS Employee2, a.department_id
FROM employees a, employees b
WHERE a.department_id = b.department_id
AND a.id <> b.id;
When to Use Self Join:
- When you need to compare rows within the same table.
- Example: Finding employees who work in the same department.
Joins in Updates
Sometimes, you may need to use joins in update queries to modify records in one table based on related data in another table.
Syntax:
UPDATE table1
INNER JOIN table2
ON table1.column = table2.column
SET table1.column = value
WHERE condition;
Example:
To update the salary of employees based on the average salary in their department:
UPDATE employees
INNER JOIN (
SELECT department_id, AVG(salary) AS avg_salary
FROM employees
GROUP BY department_id
) AS dept_avg
ON employees.department_id = dept_avg.department_id
SET employees.salary = dept_avg.avg_salary
WHERE employees.performance = 'excellent';
Joining on Multiple Columns
You can join tables on multiple columns to match records based on multiple criteria.
Syntax:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.column1 = table2.column1
AND table1.column2 = table2.column2;
Example:
To find employees and their projects based on both employee ID and project ID:
SELECT employees.name, projects.name
FROM employees
INNER JOIN projects
ON employees.id = projects.employee_id
AND employees.project_id = projects.id;
Joining Three or More Tables
Joining multiple tables is a common requirement in complex queries. You can chain multiple join operations to achieve this.
Syntax:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.column = table2.column
INNER JOIN table3
ON table2.column = table3.column;
Example:
To find employees, their departments, and their project details:
SELECT employees.name, departments.name, projects.name
FROM employees
INNER JOIN departments
ON employees.department_id = departments.id
INNER JOIN projects
ON employees.project_id = projects.id;
Conclusion
Joins are a powerful feature of SQL that allow you to combine and analyze data from multiple tables in a relational database. By understanding and using the different types of joins available in MySQL, you can perform complex queries that provide deeper insights into your data. Whether you’re performing an inner join to find exact matches, a left join to include unmatched rows, or even a self join to compare rows within the same table, mastering joins will significantly enhance your database querying capabilities.
Happy querying!
Comments