When building a SaaS (Software as a Service) application with Laravel, selecting the right packages is critical to ensure your project runs smoothly and efficiently. Laravel’s rich ecosystem provides a variety of pre-built packages that handle common tasks like authentication, billing, multi-tenancy, and file management, so developers don’t have to reinvent the wheel. However, with so many options available, it can be tricky to know which ones to choose for specific functionalities.
In this blog, we’ll guide you through some of the most useful Laravel packages for SaaS development, explaining what each package does and when to use it. Whether you’re building a multi-tenant system, managing user subscriptions, or setting up API authentication, this guide will help you pick the right tools for the job.
1. Multi-Tenancy
SaaS applications typically need to support multiple tenants (companies or users) that each have their own isolated data but share the same application instance. This is a critical aspect of SaaS architecture.
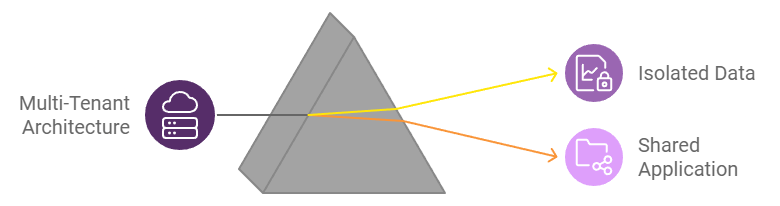
Tenancy for Laravel
- Purpose: Provides multi-tenancy support for Laravel, allowing each tenant to have separate databases or share a single database with tenant-specific data segregation.
- When to Use: Use this package when building SaaS applications where multiple customers need isolated environments or databases.Features:
- Centralized tenant management.
- Subdomain or domain-based tenant identification.
- Tenant-specific configurations.
composer require stancl/tenancy
Example configuration for database tenancy:
// config/tenancy.php
'database' => [
'central_connection' => env('DB_CONNECTION', 'mysql'),
'tenant_connection' => env('TENANT_DB_CONNECTION', 'mysql'),
]
Hyn Multi-Tenancy
- Purpose: Another popular multi-tenancy package that supports tenant separation by either subdomain or a dedicated database.
- When to Use: Use this when you need a more established package for multi-tenancy, with support for both subdomain and domain tenancy models.
composer require hyn/multi-tenant
2. Subscription and Billing Management
Handling payments and subscriptions is essential in SaaS applications. Laravel provides native solutions for subscription management and billing.
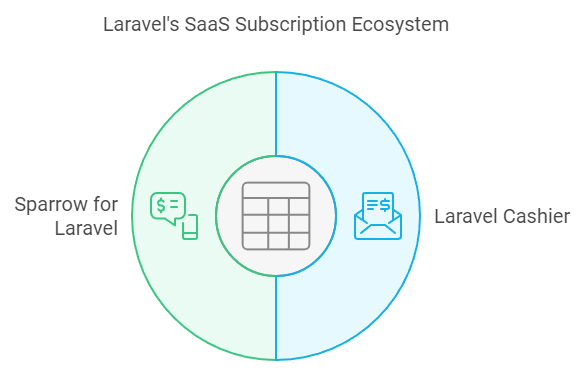
Laravel Cashier
- Purpose: Provides an easy way to integrate subscription billing with Stripe or Paddle. It handles everything from basic subscriptions to complex usage-based billing.
- When to Use: Use Cashier for managing subscription billing for your SaaS product, including plan upgrades, downgrades, metered billing, and customer invoices.
composer require laravel/cashier
Features:
- Subscriptions (monthly, yearly, etc.).
- Coupons and discounts.
- Handling plan changes (upgrades/downgrades).
- Generating invoices and handling customer payment methods.
Example:
$user = User::find(1);
$user->newSubscription('default', 'premium-plan')->create($paymentMethod);
Sparrow for Laravel
- Purpose: A package that provides subscription billing features using Braintree, Stripe, or other payment gateways.
- When to Use: Use this when you need a customizable billing solution, but prefer something other than Cashier or want to extend Cashier’s functionality.
composer require medology/sparrow
3. Authentication and User Management for SaaS
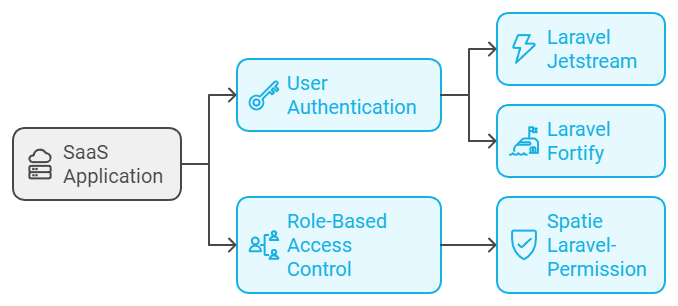
In a SaaS application, user authentication and role-based access control are key features. Laravel provides several packages to handle these functionalities efficiently.
Laravel Jetstream
- Purpose: Provides a complete user authentication package with login, registration, email verification, two-factor authentication, and team management.
- When to Use: Ideal for SaaS apps where teams need to collaborate or when you require two-factor authentication (2FA) and API tokens for users.Jetstream comes with two stacks: Livewire and Inertia.js, allowing you to choose your preferred frontend implementation.
composer require laravel/jetstream
Laravel Fortify
- Purpose: A headless authentication backend, perfect for developers who want to handle frontend logic separately.
- When to Use: Use this when you want full control over the frontend design while relying on Laravel for backend authentication (login, registration, password resets).
composer require laravel/fortify
Spatie Laravel-Permission
- Purpose: Provides role and permission management for Laravel, allowing you to assign permissions and roles to users easily.
- When to Use: Use this package to implement fine-grained user permissions for your SaaS application. For example, if you need admins, managers, and users with different access levels to different resources.
composer require spatie/laravel-permission
4. API Development for SaaS
Many SaaS platforms need APIs for external integrations or to support mobile applications. Laravel has some powerful tools for API development.
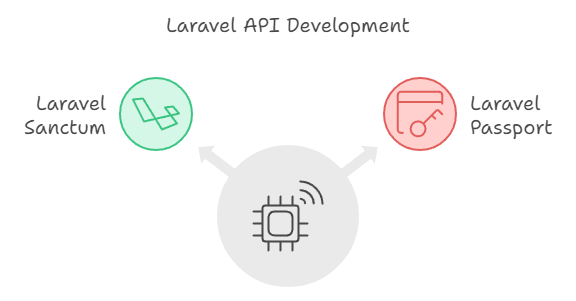
Laravel Sanctum
- Purpose: Provides a lightweight API token authentication system for SPAs (Single Page Applications), mobile applications, and basic token-based APIs.
- When to Use: Use Sanctum when you want simple API authentication for your SaaS product, especially for SPAs or internal APIs.
composer require laravel/sanctum
Example for creating an API token:
$token = $user->createToken('api-token')->plainTextToken;
Laravel Passport
- Purpose: Full OAuth2 server implementation for API authentication.
- When to Use: Use Passport for more complex API authentication scenarios where you need OAuth2, such as for third-party app integrations or when you need more advanced security.
composer require laravel/passport
php artisan passport:install
5. Notifications and Email for SaaS
Sending notifications and emails is essential in SaaS for alerts, onboarding, and subscription reminders.
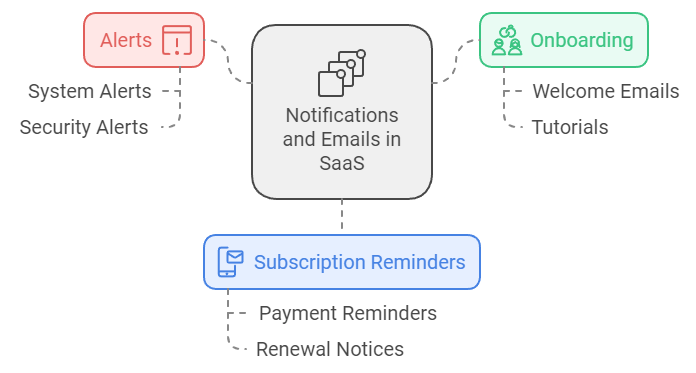
Laravel Notifications
- Purpose: Built-in system to send notifications via email, SMS, Slack, and other channels.
- When to Use: Use this when you need to send notifications such as subscription renewal reminders, invoices, or account updates.Example of sending an email notification:
Notification::send($user, new InvoicePaid($invoice));
Laravel Mailgun
- Purpose: Integrates with Mailgun for sending emails via its API.
- When to Use: Use Laravel Mailgun when you need a reliable service to handle transactional and marketing emails with advanced features like analytics and email deliverability.
composer require guzzlehttp/guzzle
6. File and Media Management for SaaS
SaaS applications often require media uploads or file management capabilities, such as user profile images or document uploads.
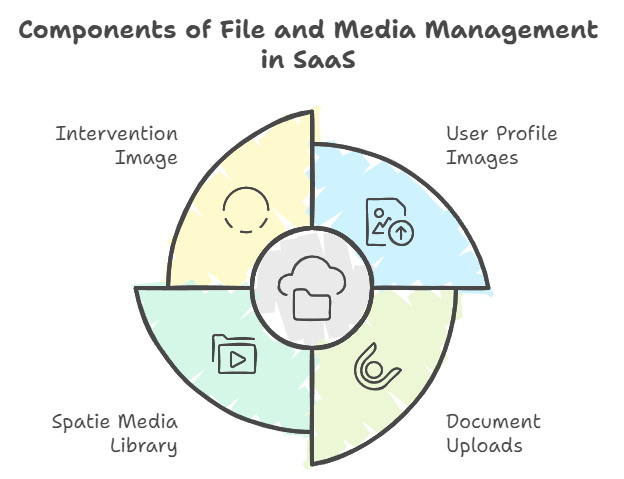
Spatie Media Library
- Purpose: Associate files (like images or documents) with Eloquent models and manage media files in a simple, structured way.
- When to Use: Use Spatie Media Library for projects where you need to store user-uploaded media files, generate image thumbnails, and manage file versions.
composer require spatie/laravel-medialibrary
Intervention Image
- Purpose: Provides an easy way to handle image manipulation such as resizing, cropping, and applying filters.
- When to Use: Use Intervention Image when your SaaS application needs to process or manipulate user-uploaded images, such as for profile pictures or content uploads.
composer require intervention/image
7. Task Scheduling and Queues for SaaS
Task scheduling and queues are important for running background jobs, handling subscriptions, sending emails, or managing data processing in a SaaS application.
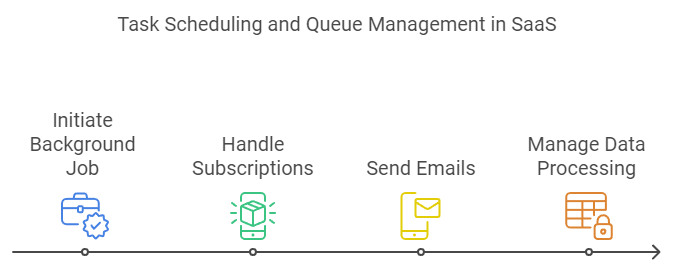
Laravel Horizon
- Purpose: A beautiful dashboard and queue manager for Redis-powered queues. It provides detailed insights into job metrics, failed jobs, and allows you to manage queue workers.
- When to Use: Use Horizon for real-time monitoring of queues in high-performance SaaS applications. It is especially useful for managing jobs like sending subscription renewal emails, processing payments, or performing data imports.
composer require laravel/horizon
Laravel Scheduler
- Purpose: Native Laravel solution for scheduling tasks such as running daily cron jobs or performing system maintenance.
- When to Use: Use Laravel Scheduler for setting up tasks like automated billing, sending daily email reports, or cleaning up expired data.Example scheduling a job in
app/Console/Kernel.php
:
protected function schedule(Schedule $schedule)
{
$schedule->command('subscriptions:renew')->daily();
}
8. Security for SaaS
Security is crucial for any SaaS application, especially when dealing with sensitive customer data and payments.
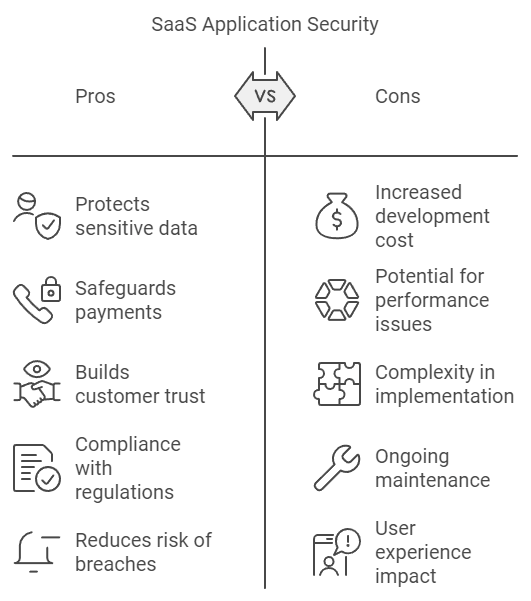
Laravel Honeypot
- Purpose: Prevents spam bot submissions by adding a hidden honeypot field in your forms.
- When to Use: Use this to protect your SaaS application from spam bots without needing CAPTCHAs.
composer require spatie/laravel-honeypot
Spatie Laravel Activitylog
- Purpose: Logs model changes, user activities, and actions, which can be useful for auditing and monitoring user interactions.
- When to Use: Use this package to track user activities, such as changes in billing information, role assignments, or updates to user profiles.
composer require spatie/laravel-activitylog
Conclusion
Building a SaaS application with Laravel is greatly simplified by leveraging the right packages for each specific task. Laravel’s ecosystem provides a wide range of packages that are both powerful and easy to integrate, enabling you to build scalable, secure, and maintainable SaaS applications.
Here’s a quick summary of the recommended packages:
Task | Recommended Package |
---|---|
Multi-Tenancy | Tenancy for Laravel, Hyn Multi-Tenancy |
Subscription & Billing | Laravel Cashier, Sparrow |
Authentication & User Management | Laravel Jetstream, Spatie Permissions |
API Authentication | Laravel Passport, Sanctum |
Notifications & Email | Laravel Notifications, Mailgun |
File & Media Management | Spatie Media Library, Intervention Image |
Task Scheduling & Queues | Laravel Horizon, Scheduler |
Security | Spatie Activity Log, Laravel Honeypot |
By carefully selecting the right tools for each aspect of your SaaS platform, you’ll be able to build robust and scalable applications faster, without compromising on features or security.
Comments