Node.js is an open-source, cross-platform runtime environment that allows you to run JavaScript code outside of a web browser. Traditionally, JavaScript was used only on the client side (front-end) to make web pages interactive. But with Node.js, you can use JavaScript on the server side (back-end) as well.
In simple terms, Node.js lets you build server applications using JavaScript. It uses Google’s V8 JavaScript engine (the same engine that powers Google Chrome) to execute code, which makes it very fast.
Why Use Node.js?
Here are some reasons why developers choose Node.js:
- Single Language for Both Front-end and Back-endIf you already know JavaScript for front-end development, you don’t need to learn a new language for back-end development. This makes the development process smoother and faster.
- Asynchronous and Non-BlockingNode.js uses an event-driven, non-blocking I/O model. This means it can handle multiple requests at the same time without waiting for one task to finish before starting another. This is ideal for applications that require high performance and scalability.
- Large Community and EcosystemNode.js has a vast community of developers. There’s a wealth of open-source libraries and modules available through npm (Node Package Manager) that you can use in your projects, saving you time and effort.
- High PerformanceBecause Node.js uses the V8 engine, it compiles JavaScript code into machine code, which makes execution very fast.
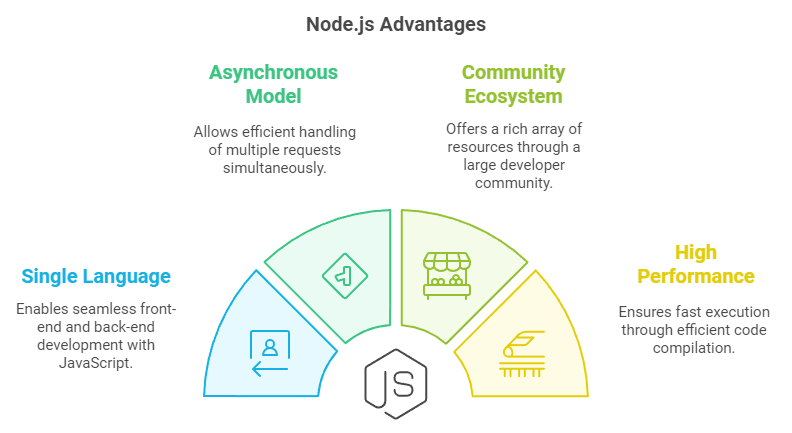
How Does Node.js Work?
At its core, Node.js uses an event-driven architecture. Here’s how it works:
- Single-Threaded Event LoopNode.js operates on a single thread using an event loop. This loop listens for events and triggers callback functions when an event occurs.
- Non-Blocking I/O OperationsInput/output operations (like reading files, making network requests) are handled asynchronously. Instead of waiting for an operation to complete, Node.js moves on to handle other tasks. When the operation finishes, it triggers an event to let Node.js know.
- Callbacks and PromisesAsynchronous operations often use callbacks or promises to handle the result once it’s available.
Key Components of Node.js
- V8 JavaScript EngineThis is the engine developed by Google for Chrome. It compiles JavaScript into machine code, making execution fast.
- Libuv LibraryLibuv is a C library that provides the event loop and asynchronous I/O capabilities to Node.js.
- Node.js Standard LibraryNode.js comes with a set of built-in modules (like
http
,fs
,path
, etc.) that provide essential functionalities. - npm (Node Package Manager)npm is the default package manager for Node.js. It hosts thousands of packages that you can install and use in your projects.
Types of Web Applications Using Node.js
Node.js is versatile and powers a wide range of web applications across various categories. Here are some types of web apps that use Node.js:
- Real-Time Applications
- Chat Applications: Node.js is perfect for chat apps that require instant messaging, like WhatsApp Web or Slack.
- Collaboration Tools: Apps like Trello and Google Docs use Node.js to enable real-time collaboration between users.
- Single Page Applications (SPAs)
- Dynamic Web Apps: Applications like Gmail and Twitter use SPAs to provide a seamless user experience without frequent page reloads.
- Streaming Applications
- Media Streaming Services: Platforms like Netflix use Node.js to handle streaming data efficiently.
- Audio and Video Streaming: Real-time streaming apps rely on Node.js for its ability to process data quickly.
- Microservices Architecture
- Modular Web Applications: Companies like Walmart use Node.js to build microservices, making their applications more scalable and easier to manage.
- API Servers
- RESTful APIs: Node.js is commonly used to build APIs that serve data to client applications.
- GraphQL APIs: For applications that need flexible data queries, Node.js works well with GraphQL.
- E-commerce Applications
- Online Marketplaces: E-commerce platforms like eBay use Node.js to handle high traffic and real-time data updates.
- Social Media Applications
- Networking Platforms: LinkedIn uses Node.js for its mobile app server due to its scalability and performance.
- Internet of Things (IoT) Applications
- Sensor Data Processing: Node.js can handle multiple device connections and process data in real-time, making it ideal for IoT solutions.
- Command-Line Tools
- Automation Scripts: Developers use Node.js to create command-line tools that automate repetitive tasks.
- Enterprise Applications
- Business Solutions: Large-scale applications in enterprises use Node.js for its efficiency and scalability.
- Content Management Systems (CMS)
- Custom CMS Platforms: Node.js can be used to build customizable CMS systems tailored to specific needs.
- Real-Time Dashboards
- Monitoring Tools: Applications that display live data analytics, like stock market dashboards, use Node.js for instant updates.
Common Use Cases for Node.js
- Web Servers and APIsNode.js is excellent for building web servers and APIs. Frameworks like Express.js simplify the process.
- Real-Time ApplicationsApplications that require real-time communication (like chat apps, online games) benefit from Node.js’s event-driven nature.
- Microservices ArchitectureNode.js is suitable for building microservices, allowing you to split your application into smaller, manageable pieces.
- Streaming ApplicationsNode.js can handle data streams efficiently, making it ideal for streaming applications.
- Command-Line ToolsYou can use Node.js to create CLI tools and scripts.
Advantages of Node.js
- Fast and EfficientNode.js is fast due to its non-blocking I/O and the V8 engine.
- ScalableThe event-driven architecture allows Node.js to handle many connections simultaneously.
- Full-Stack JavaScriptUsing the same language on both the front-end and back-end simplifies development.
- Large EcosystemWith npm, you have access to thousands of packages that can add functionality to your application.
- Active CommunityA large community means plenty of tutorials, resources, and support.
Disadvantages of Node.js
- Single-Threaded LimitationsNode.js runs on a single thread, which means CPU-intensive tasks can block the event loop and degrade performance.
- Callback HellHeavy reliance on callbacks can make code difficult to read and maintain. However, this can be mitigated using Promises and async/await.
- Maturity of ModulesWhile there are many modules available, not all are well-documented or maintained.
- Asynchronous Programming ComplexityFor developers new to asynchronous programming, understanding how to write and debug asynchronous code can be challenging.
Comparing Node.js with Other Technologies
Understanding how Node.js stacks up against other server-side technologies can help you make informed decisions for your projects. Here’s a comparison of Node.js with some popular back-end technologies:
Node.js vs. PHP
- Language
- Node.js: Uses JavaScript, allowing for full-stack development with a single language.
- PHP: A server-side scripting language specifically designed for web development.
- Performance
- Node.js: Generally offers better performance due to its non-blocking, event-driven architecture.
- PHP: Traditionally slower because it handles each request with a separate process.
- Concurrency
- Node.js: Handles concurrency using a single-threaded event loop, making it efficient for I/O-heavy applications.
- PHP: Relies on multi-threading, which can consume more resources under heavy load.
- Use Cases
- Node.js: Ideal for real-time applications, APIs, and microservices.
- PHP: Commonly used for content-heavy websites and CMS platforms like WordPress.
Node.js vs. Python
- Language
- Node.js: JavaScript runtime for server-side applications.
- Python: A general-purpose programming language known for its readability and extensive libraries.
- Performance
- Node.js: Better suited for applications requiring high concurrency.
- Python: Slower in handling multiple threads but excels in CPU-intensive tasks.
- Use Cases
- Node.js: Great for web applications, real-time services, and event-driven servers.
- Python: Preferred for data analysis, machine learning, and scripting.
- Community and Libraries
- Both have large communities and a rich set of libraries, but they cater to different domains.
Node.js vs. Java
- Language
- Node.js: JavaScript, dynamically typed and interpreted.
- Java: Statically typed, compiled language.
- Performance
- Node.js: Excels in handling a large number of simultaneous connections with minimal overhead.
- Java: Offers high performance for enterprise-level applications, especially when dealing with complex computations.
- Use Cases
- Node.js: Suitable for microservices, lightweight and scalable applications.
- Java: Often used in large-scale enterprise applications, Android app development.
Node.js vs. Ruby on Rails
- Framework
- Node.js: A runtime environment; you need to use frameworks like Express.js.
- Ruby on Rails: A full-stack web application framework.
- Performance
- Node.js: Generally faster due to non-blocking I/O.
- Ruby on Rails: Can be slower but provides many built-in features that speed up development time.
- Community
- Both have active communities, but Ruby on Rails is known for strong conventions and a “Rails way” of doing things.
When to Choose Node.js
- Real-Time ApplicationsIf your application requires real-time updates, Node.js is a strong candidate.
- Single Language DevelopmentWhen you want to use JavaScript across the entire stack.
- High ConcurrencyFor applications that need to handle many simultaneous connections efficiently.
When to Consider Other Technologies
- CPU-Intensive TasksIf your application performs heavy computations, a multi-threaded language like Java or a compiled language like Go might be better.
- Specific Domain NeedsFor tasks in data science or machine learning, Python offers extensive libraries and tools.
Conclusion
Node.js has revolutionized server-side development by allowing developers to use JavaScript on the back-end. Its asynchronous, event-driven architecture makes it ideal for building scalable and high-performance applications.
Whether you’re developing a simple website, a complex web application, or a real-time chat system, Node.js provides the tools and community support to help you succeed.
By understanding the basics, comparing it with other technologies, and continually learning, you can harness the full potential of Node.js in your projects.
Comments