Welcome to the world of programming! If you’re new here, you’re probably trying to get a grip on some of the basic concepts that make up the foundation of coding. One such essential concept is data types. In this blog, we’ll dive into what data types are, why they’re important, and how they work in different programming languages. Whether you’re just starting or need a quick refresher, this guide is tailored just for you.
What Are Data Types?
Imagine you’re organizing a cupboard. You have different shelves for books, clothes, and dishes. In programming, data types work like these shelves. They help categorize your data (like numbers or letters) so the computer knows how to store and use them correctly.
- Think of Numbers and Letters: Just like how you separate books and dishes, the computer separates numbers and letters using data types.
- Instructions for the Computer: Data types tell the computer, “This is a number” or “This is a text”, so it knows what to do with them.
- Organizing Your Code: Using data types keeps your code neat and understandable, just like a well-organized cupboard.
In short, data types are like labels that help computers understand and organize different kinds of data in your code.
Why Are Data Types Important?
Data types are crucial in programming for several key reasons:
- Accuracy: They ensure that operations on data are performed correctly. For instance, knowing whether a value is a number or a text string is essential for accurate calculations or manipulations.
Example - When performing arithmetic operations, knowing if a value is an integer or a float is crucial. For example, 5 / 2
is 2.5
(float) and not just 2
(integer). This distinction ensures precise calculations.
- Efficiency: Different data types use different amounts of memory. By specifying the correct type, programs use resources more efficiently, making them faster and more reliable.
Example: Storing a small number in an 'int' type uses less memory than storing it in a 'long' type. If you know your number won’t exceed a certain range, choosing 'int' over 'long' makes your program more memory-efficient.
- Preventing Errors: When you use the right data type, you reduce the risk of errors in your code. For example, trying to divide a word by a number doesn’t make sense – data types help prevent such mix-ups.
Example: If you try to perform a mathematical operation between a string and a number (like "apple" + 5
), the program will throw an error. Proper data types prevent such incompatible operations.
- Clarity in Code: Data types make your code clearer not just to the computer, but also to anyone else (or even yourself) reading your code later. They give a quick understanding of what kind of data is being handled.
Example: When you see a variable declared as a 'boolean', such as isStudent = true
, it's immediately clear that this variable represents a true/false condition, aiding in understanding the code's purpose.
In essence, data types are like the rules of a game – they make sure everything in your program plays out smoothly and as expected.
Types of Data Types
In programming, data types are broadly categorized into two main types: Primitive and Non-Primitive. Here’s a brief overview of each:
1. Primitive Data Types
Primitive data types are the most basic forms of data in programming and are built directly into the language. They represent simple, fundamental values like numbers and characters.
Types of Primitive Data Types
- Integer
- Stores whole numbers, like 5, -3, or 42.
- Memory Usage: Typically 4 bytes (may vary based on the programming language and system architecture).
- Float (or Double)
- Holds numbers with fractional parts, such as 3.14 or -0.001.
- Memory Usage: A Float often takes up 4 bytes, while a Double usually requires 8 bytes.
- Character
- Contains single characters like ‘A’, ‘z’, or ‘4’.
- Memory Usage: Generally 1 byte, enough to represent a character in the ASCII table.
- Boolean
- Represents true or false values, essential in control flow and conditional statements.
- Memory Usage: Typically 1 byte, though it’s simply used to represent a 0 or 1 value.
Memory Management for Primitive Data Types
- Fixed Size and Stack Allocation: Primitive data types have a predetermined, fixed memory size. For example, an
Integer
typically takes up 4 bytes, and aCharacter
usually occupies 1 byte. Because of their fixed size, these types are often stored in the stack memory, which is known for fast access and efficient memory use. - Efficient Memory Usage: Since the size of these types is known at compile time and doesn’t change at runtime, they are highly memory-efficient. This makes operations on primitive data types fast and reduces the program’s overall memory footprint.
- Automatic Memory Management: Memory for primitive data types is managed automatically by the system. When a function that uses these types is called, the necessary memory is allocated on the stack, and when the function exits, this memory is automatically freed.
Primitive Data Types in different programming language
1. Integer
- JavaScript: JavaScript doesn’t have a specific ‘integer’ type; it represents all numbers as floating-point values. It uses 64-bit format IEEE 754 numbers, which typically occupy 8 bytes.
- Python: Python’s integers have a variable size. They can use 28 bytes or more, depending on the magnitude of the number.
- PHP: In PHP, an integer’s size is platform-dependent. On a 32-bit system, integers typically use 4 bytes, while on a 64-bit system, they use 8 bytes.
- Java: In Java, an
int
is always 4 bytes.
2. Float (or Double)
- JavaScript: As mentioned, JavaScript uses 64-bit floating-point format for all numbers, which typically occupies 8 bytes.
- Python: Python’s
float
type corresponds to a C double and is typically 8 bytes. - PHP: A float in PHP typically uses 8 bytes, similar to a double in C.
- Java: In Java, a
float
occupies 4 bytes, while adouble
uses 8 bytes.
3. Character
- JavaScript: JavaScript doesn’t have a specific character type and represents them as strings. A single UTF-16 character in JavaScript usually occupies 2 bytes.
- Python: In Python 3.x, a character (string of length 1) is represented in Unicode and typically uses 1 to 4 bytes.
- PHP: PHP characters are part of strings, and a single ASCII character uses 1 byte.
- Java: In Java, a
char
uses 2 bytes, as it is stored in UTF-16.
4. Boolean
- JavaScript: In JavaScript, a boolean value doesn’t have a fixed size but is often represented using 4 bytes internally for optimization purposes.
- Python: Python’s boolean values (
True
andFalse
) are a subclass of integers and are typically represented using 4 bytes. - PHP: PHP uses 1 byte for boolean values.
- Java: In Java, a
boolean
typically uses 1 byte, but its actual size is not precisely specified in the language specification.
2. Non-Primitive Data Types
These are more complex data types that are derived from primitive data types. They can store multiple values or complex data.
- String: A sequence of characters, like “Hello, world!” or “123abc”.
- Array: A collection of elements, typically of the same primitive data type, like an array of integers [1, 2, 3].
- Object (in Object-Oriented Programming): Represents a complex entity with properties and methods. For instance, a ‘Car’ object might have properties like color, model, and methods like start() or stop().
Memory Management of Non-Primitive Data Types
- String
- Memory Allocation: Strings in programming languages are typically allocated in the heap memory because their size can vary.
- Memory Usage:
- JavaScript/Python/PHP: These languages handle strings dynamically, adjusting the memory allocation as the string grows or shrinks. The memory used depends on the length of the string and character encoding.
- Java: Strings in Java are objects and are stored in the heap. Java uses a concept called ‘String Pool’ for memory efficiency, especially when the same string is used multiple times.
- Array
- Memory Allocation: Arrays are also allocated in heap memory, as their size can be larger and more flexible than primitive data types.
- Memory Usage:
- JavaScript: JavaScript arrays are dynamic and can store different types of elements. They use more memory than a typical static array because of this flexibility.
- Python: Lists in Python (similar to arrays) have an overhead for each element, which is larger than the memory required for the element itself.
- PHP: Arrays in PHP are actually ordered maps and are more memory-intensive compared to simple, static arrays.
- Java: In Java, arrays are objects. They have a fixed size, and memory usage depends on the type of elements and the array size.
- Object (in Object-Oriented Programming)
- Memory Allocation: Objects are stored in heap memory, which allows for more complex data structures.
- Memory Usage:
- JavaScript/Python/PHP: These languages dynamically allocate memory for objects based on the properties and methods they contain. The memory used depends on the number and type of properties.
- Java: In Java, every object has some memory overhead for the object’s metadata (like type information) in addition to the memory used by its properties.
Dynamic Nature and Garbage Collection
- Dynamic Memory Allocation: Unlike primitive types, non-primitive types often require memory that can grow or shrink at runtime. This dynamic allocation happens in heap memory.
- Garbage Collection: Languages like JavaScript, Python, and Java use garbage collectors to automatically manage memory. Garbage collectors periodically scan for objects that are no longer in use and reclaim their memory, reducing memory leaks and overflow issues.
Impact on Performance
- Flexibility vs. Efficiency: Non-primitive types offer great flexibility, such as resizable arrays or strings. However, this comes at the cost of increased memory usage and potentially slower performance due to the overhead of dynamic memory management and garbage collection.
- Manual Management in Some Languages: In languages that don’t automatically manage memory, like C++, programmers need to manually allocate and deallocate memory for complex data types, which requires careful handling to avoid memory leaks.
Choosing the Right Data Type
Selecting the appropriate data type is critical in programming, as it can significantly impact the efficiency, readability, and functionality of your code. Let’s explore some scenarios and examples to illustrate the importance of choosing the right data type:
1. Efficiency in Resource Usage
- Scenario: Storing a large list of ages of individuals.
- Right Choice: An
integer
data type is suitable because ages are whole numbers. Using afloat
ordouble
would be inefficient as these types consume more memory and are meant for numbers with fractional parts. - Example: In Python, you’d use
ages = [25, 30, 45]
instead ofages = [25.0, 30.0, 45.0]
.
2. Ensuring Accuracy
- Scenario: Calculating the average of a set of measurements.
- Right Choice: A
float
ordouble
data type should be used to handle the possibility of fractional values and maintain precision. - Example: In Java, you might declare
double average = 34.67
instead ofint average = 34
.
3. Memory Optimization
- Scenario: Representing a status flag that indicates whether a user is online or offline.
- Right Choice: A
boolean
data type is ideal as it only needs to represent two states: true (online) or false (offline). - Example: In JavaScript, you could use
let isOnline = true
rather than storing this information as aString
orNumber
.
4. Handling Textual Data
- Scenario: Storing and manipulating names or addresses.
- Right Choice: A
string
data type is appropriate for textual data. - Example: In PHP, you would use
$name = "John Doe"
for storing a name.
5. Complex Data Structures
- Scenario: Keeping track of a list of items along with their quantities and prices.
- Right Choice: An
array
orobject
(ordictionary
in Python) to store complex, multi-attribute data. - Example: In Python, you could use
items = [{"name": "apple", "quantity": 10, "price": 0.30}, {"name": "banana", "quantity": 5, "price": 0.20}]
.
6. Space-Sensitive Applications
- Scenario: Developing an application for a memory-constrained environment, like an embedded system.
- Right Choice: Choose the smallest data types that can comfortably handle the expected range of values. For instance, using
byte
orshort
instead ofint
if the values are small. - Example: In C++, you might use
uint8_t age = 25
where the age will not exceed 255.
In each scenario, the chosen data type aligns with the needs of the data being represented — whether it’s for optimizing memory, maintaining precision, or handling complex data structures. Making the right choice in data types not only makes your program more efficient and effective but also enhances its clarity and maintainability.
Data Types in Different Programming Languages
Different programming languages have their unique ways of handling data types. Let’s look at some examples in JavaScript, Python, PHP, and Java to understand how each of these languages deals with common data types.
JavaScript
- Dynamic Typing: JavaScript is a dynamically typed language, meaning variables can hold any data type and can change type.
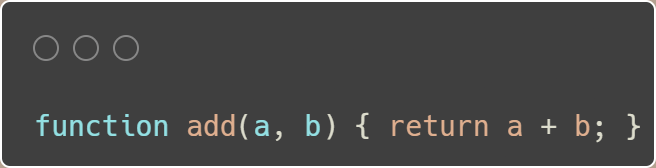
- Primitive Types: Number, String, Boolean, Undefined, Null, Symbol (ES6).
- Non-Primitive: Object (including Arrays, Functions, and more).
Python
- Dynamic and Strong Typing: Python is dynamically typed but also strongly typed, meaning while you don’t need to declare data types explicitly, once a data type is set, it tends to enforce its rules.
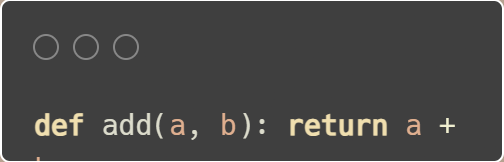
- Primitive Types: In Python, types like integers, floats, and strings are considered basic types but are technically objects.
- Non-Primitive: Lists, Tuples, Sets, Dictionaries, and more.
PHP
- Loosely Typed: PHP is a loosely typed language, allowing variable types to be changed dynamically.
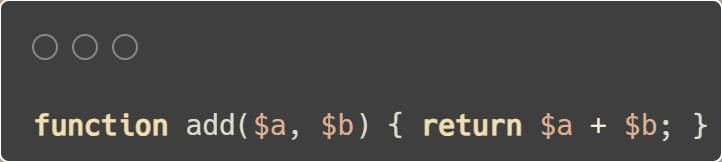
- Primitive Types: Integer, Float, String, Boolean.
- Non-Primitive: Array, Object, and special types like Resource and NULL.
Java
- Static Typing: Java requires explicit data type declarations, making it statically typed. Once a variable’s data type is set, it cannot change.

· Primitive Types: byte, short, int, long, float, double, char, boolean.
· Non-Primitive: Arrays, Classes, Interfaces.
Conclusion
In conclusion, understanding data types and their management across different programming languages is crucial for effective coding. Here are the key points we’ve covered:
Primitive vs. Non-Primitive Data Types:
- Primitive data types are simple, basic types like integers and booleans.
- Non-primitive data types are more complex, like strings and objects, capable of storing multiple values or intricate data structures.
Memory Management:
- Primitive data types generally have fixed memory allocation and are stored in stack memory.
- Non-primitive data types often involve dynamic memory allocation in heap memory and can have a more significant memory footprint.
Choosing the Right Data Type:
- The choice of data type affects resource efficiency, accuracy, and overall performance.
- Proper selection is vital for memory optimization and avoiding errors.
Data Types in Various Languages:
- Languages like JavaScript, Python, PHP, and Java have distinct ways of handling data types, reflecting their paradigms and syntax.
- JavaScript and Python offer dynamic typing, PHP is loosely typed, and Java requires explicit type declarations.
Examples and Scenarios:
- Code snippets and scenarios provide practical insights into how different data types are used and managed in various programming contexts.
By keeping these key points in mind, you can write more efficient, readable, and maintainable code, effectively leveraging the strengths of different data types and programming languages.
Comments